For developers preparing for technical interviews, mastering Angular module architecture is essential. This core aspect of Angular development shapes how web applications are structured, maintained, and scaled. Employers often evaluate candidates on concepts like Angular expressions, lazy loading, and source code organization. Mistakes in answering these Angular interview questions can detract from technical expertise, making preparation critical for securing top roles.
At Aloa, we deliver high-quality software solutions using cutting-edge technologies. With a robust project management framework, we connect clients to vetted development teams skilled in advanced frameworks like Angular. Our transparent project dashboard and US-based Product Owners ensure clients optimize their development strategies with confidence.
To support developers, we’ve curated a list of 12+ essential Angular and Angular JS interview questions on module architecture. This guide offers practical insights and examples on topics like the purpose of NgModule, resolving circular dependencies, and implementing lazy loading, helping developers confidently showcase their skills and tackle complex challenges.
Let’s get started!
Core Concepts of Angular Module Architecture
Angular's modular architecture is key to building scalable, maintainable applications by organizing functionality into cohesive blocks. A common topic in Angular interview questions it tests a developer's ability to streamline development, ensure code reuse, and optimize performance—essential skills for designing efficient, modular software systems.
Data binding is a core concept in Angular that refers to the communication between the component class and the HTML template. It allows the synchronization of the component property and the view, ensuring that the data in the application stays up to date. There are different types of data binding in Angular, such as string interpolation, property binding (using square brackets), event binding, and two-way binding (using ngModel).
String interpolation uses curly braces ({{ }}) to bind typescript code expressions to the HTML template, allowing dynamic content to be displayed on web pages.
What is an Angular module, and why is it important?
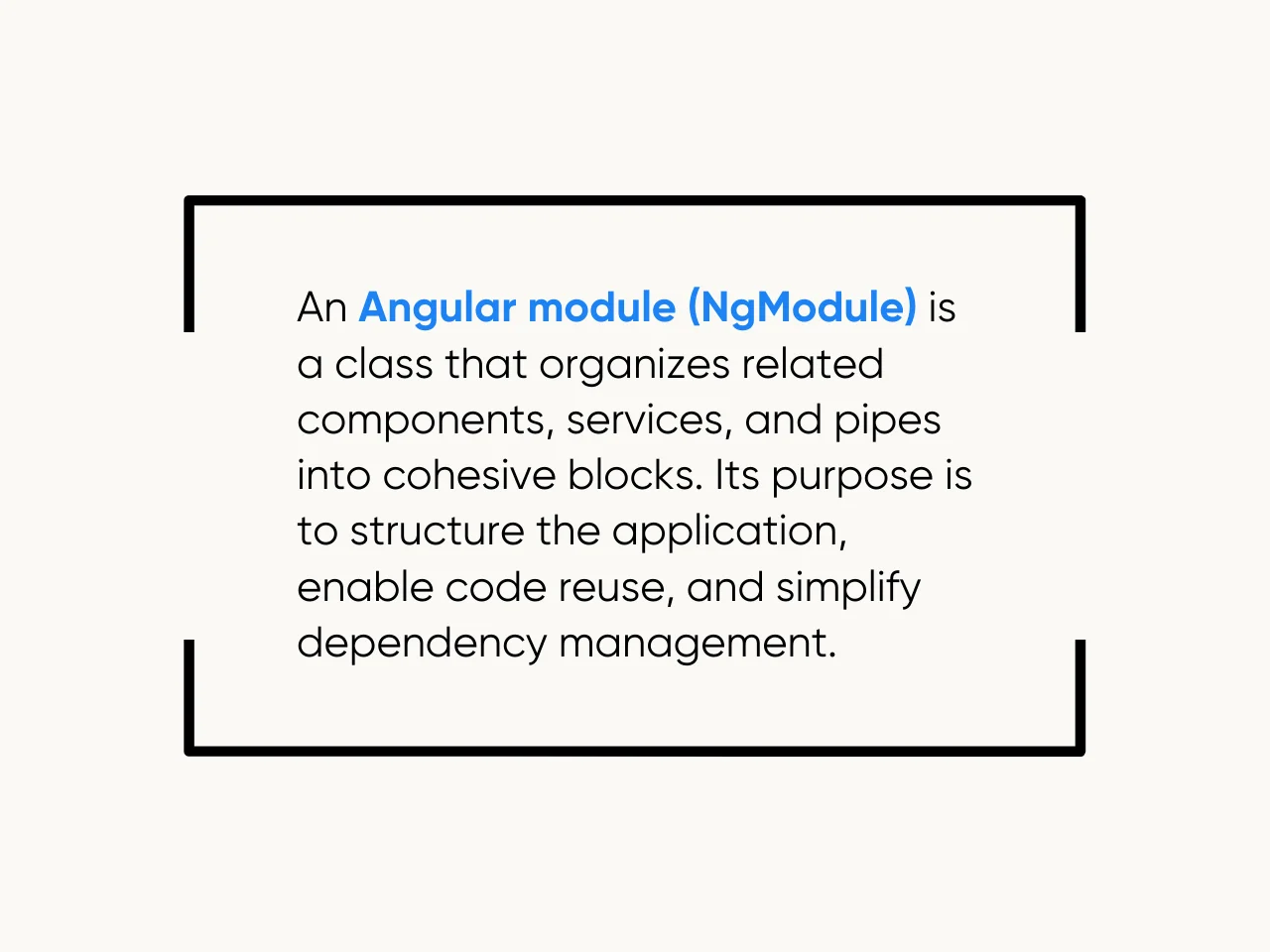
An Angular module (NgModule) is a class that organizes an application into cohesive blocks by grouping related components, directives, services, and pipes. This approach improves code maintainability, reusability, and testability.
The purpose of NgModule is to structure an Angular application into feature-specific modules. It supports reusability by allowing code to be shared across various parts of the application. It also enhances scalability for managing large applications and simplifies service management through Angular's dependency injection system.
Metadata Fields in NgModule:
- Declarations: Declares the components, directives, and pipes that belong to the module.
- Imports: Specifies other modules required for this module to function.
- Providers: Registers services available for dependency injection throughout the module.
- Bootstrap: Identifies the root component to bootstrap at application start.
Example Configuration of an Angular Module:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { HeaderComponent } from './header.component';
@NgModule({
declarations: [AppComponent, HeaderComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
How do you differentiate between root and feature modules?
The root module, typically named AppModule or app module, is the entry point of an Angular application. It initializes the application by bootstrapping the root component, usually AppComponent, and importing core modules like BrowserModule. Think of it as the foundation of the app—it sets up global configurations and services that are accessible throughout the application. Since the root module is responsible for starting the app, there can only be one root module.
On the other hand, feature modules are modularized sections of the application designed to encapsulate specific functionalities, such as user management, dashboards, or reports. Feature modules group related components, services, and directives into a cohesive block, making them reusable and easier to manage.
Unlike the root module, feature modules typically import CommonModule instead of BrowserModule. A key advantage of feature modules is their support for lazy loading, which loads them only when they are needed, reducing the application’s initial load time and improving performance.
Best practices include:
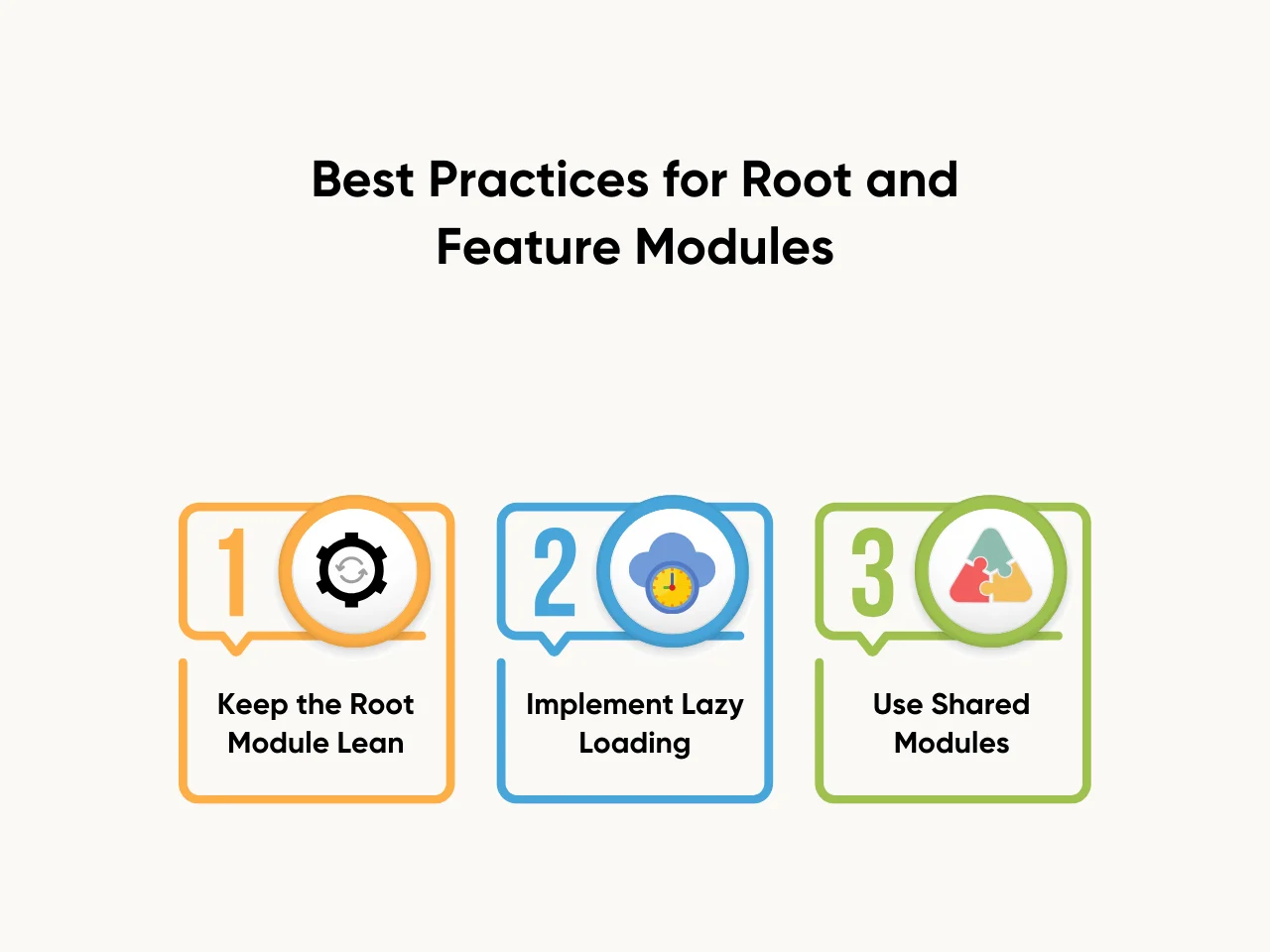
- Keep the Root Module Lean: Delegate functionality to feature modules to avoid overloading the root module.
- Implement Lazy Loading: Use lazy loading for feature modules to optimize performance.
- Use Shared Modules: Group reusable components and utilities in shared modules to reduce redundancy across feature modules.
What are shared modules, and how are they used?
In Angular, shared modules are a cornerstone of efficient application design, enabling the reuse of common components, directives, and pipes across multiple feature modules. They streamline application architecture by reducing code duplication and ensuring consistency in functionality and style across the app.
The main purpose of a shared module is to house reusable logic and UI elements that multiple parts of the application rely on. These can include UI components like buttons or headers, directives for common behaviors, and pipes for formatting data. Instead of duplicating these elements across feature modules, a shared module consolidates them in one place, improving maintainability and reducing redundancy.
In answering interview questions on Angular such as this, remember the advantages of shared modules:
- Consistency: Ensures a unified design and behavior across the application.
- Efficiency: Reduces development time by avoiding repeated code.
- Ease of Maintenance: Centralizes updates to shared functionality, ensuring changes propagate without manual adjustments in multiple modules.
To create a shared module, you declare and export reusable components, directives, and pipes. You also import foundational Angular modules like CommonModule to enable essential Angular directives and features.
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { CustomButtonComponent } from './custom-button.component';
import { HeaderComponent } from './header.component';
@NgModule({
declarations: [CustomButtonComponent, HeaderComponent], // Reusable UI components
exports: [CustomButtonComponent, HeaderComponent], // Make these available to other modules
imports: [CommonModule] // Import essential Angular features
})
export class SharedModule { }
This SharedModule allows components like CustomButtonComponent and HeaderComponent to be reused in any feature module by simply importing SharedModule.
When using shared modules, a critical best practice is not to import them into the root module (AppModule). Doing so can lead to circular dependencies, which may cause runtime errors and make debugging more difficult. Instead, restrict shared modules to feature modules that require access to their components or functionalities.
Dependency Injection and Providers in Modules
Angular's DI system also works closely with lifecycle hooks to manage the creation and destruction of instances, thereby ensuring that services are properly injected and that asynchronous operations, such as fetching application data, are effectively managed. A frequent topic in Angular interview questions, the change detection mechanism ensures that any changes in the data model are reflected in the user interface.
Angular also uses decorators such as property decorators, method decorators, and class decorators to provide metadata that informs Angular how to process classes, methods, or properties. These decorators are integral to understanding how Angular's dependency injection system works.
How does Angular handle dependency injection in modules?
Angular's DI system uses a hierarchical injector to manage service instances based on their declared scope. The root injector provides services globally, while feature modules, lazy-loaded modules, and components can have their own injectors, ensuring isolation and lifecycle control.
Providers configure how Angular's injector creates and delivers services. Declared in modules, components, or directives, they define a service's scope and lifetime. The providedIn property in the @Injectable decorator specifies where the service is available in the application.
In answering Angular interview questions, remember these scopes:

- Root-Level Providers: Declaring a service with providedIn: 'root' ensures that it is globally available as a singleton, meaning only one instance of the service exists across the entire application. This approach is efficient for services like authentication or API calls that need a global state.
- Module-Level Providers: Declaring a service in a module’s providers array scopes the service to that module. This is useful when a service is required only for specific functionalities encapsulated within that module.
- Component-Level Providers: Defining a service in a component’s providers array creates a new instance of the service for that component and its child components. This approach is ideal for services with localized state management needs.
In Angular, declaring a service with providedIn: 'root' ensures it is a singleton, meaning a single instance of the service is created and shared across the entire application. This configuration registers the service with the root injector, making it globally accessible without needing to add it to a module’s providers array.
An authentication service (AuthService) that tracks user login status is a common use case for singleton services. By using providedIn: 'root', the service maintains a consistent state (e.g., logged in or logged out) across the app, ensuring all modules and components access the same instance.
What is the difference between forRoot() and forChild() methods?
In Angular, the forRoot() and forChild() methods are used to configure modules, particularly when setting up routing. They serve different purposes and are crucial for ensuring proper modular functionality in an Angular application.
The forRoot() method is used to configure and provide services or dependencies at the root module level. It ensures that these configurations are instantiated once and shared globally across the application.
- Purpose: To set up global services or single-instance configurations.
- Example Use Case: Configuring the main routes of an application with RouterModule.forRoot(routes) ensures that the application-level router is initialized properly.
The forChild() method is used when feature modules need to add their own routes or use a shared service without re-instantiating it. It helps avoid conflicts and ensures modular routing works seamlessly.
- Purpose: To define routes or dependencies specific to feature modules.
- Example Use Case: A feature module for a dashboard might use RouterModule.forChild(dashboardRoutes) to define its own routing while integrating into the main routing system.
In Angular, RouterModule.forChild(routes) is used within feature modules to define their specific routes while seamlessly integrating with the application's main routing system. This approach ensures that each feature module manages its own routing logic independently of the root routing configuration.
For instance, a feature module like DashboardModule might define routes for components such as a dashboard home page or settings page. Using forChild(), these routes are encapsulated within the feature module, maintaining modularity and preventing conflicts with the global router, which is configured using RouterModule.forRoot(routes).
Feature modules can contribute to the overall application routing without duplicating or interfering with the global routing system by employing forChild(). This design simplifies scaling, testing, and maintaining individual modules in larger applications.
How do you share services across multiple modules?
Sharing services effectively in Angular requires strategies that ensure reusability and prevent duplication, which can lead to unexpected behavior. Here are the best practices to remember when answering such Angular interview questions:
The most efficient way to share services across multiple modules is to provide them at the root level. This is achieved by using the providedIn: 'root' option in the service's @Injectable decorator. When a service is provided in the root injector, Angular creates a singleton instance accessible throughout the application.
- Benefits: Ensures a single instance of the service is shared across all modules and components. This approach avoids redundancy and improves performance.
- Example Use Case: Services like AuthService or ApiService, which manage global states or operations, are ideal candidates for root-level provision.
To prevent creating multiple instances of a service, do not include the service in the providers array of feature modules. Doing so limits the service scope to that module and creates a new instance each time the module is loaded.
If a service needs to be shared, ensure it is not explicitly added to a feature module's providers. Instead, rely on the global instance provided by the root injector.
Advanced Module Architecture Concepts
Angular's module system offers advanced techniques to optimize performance and scalability, especially in larger applications. Developers can ensure that applications remain efficient by leveraging concepts like lazy loading and modular routing, even as they grow in complexity.
What is lazy loading, and how is it implemented in Angular?
Lazy loading in Angular is a performance optimization strategy where feature modules are loaded only when they are needed, instead of being loaded at the application’s startup. This reduces the initial load time, improving the application's responsiveness and scalability, especially for large applications.
If asked in Angular interview questions, the benefits of Lazy Loading include:

- Faster Initial Load: Only the essential modules are loaded at startup.
- Improved Performance: Reduces memory usage by loading modules on demand.
- Better Maintainability: Encourages modular design, simplifying application management.
If asked in Angular interview questions, the implementation steps are:
1. Define Lazy-Loaded Routes in RouterModule
Lazy-loaded routes are specified using the loadChildren property in the application's routing configuration. This property points to the module that should be loaded when the route is accessed.
2. Use the loadChildren Property
The loadChildren syntax dynamically imports the feature module. This ensures that Angular fetches and loads the module only when its route is visited. For example:
- In the root routing file, configure routes with loadChildren for lazy loading.
- Avoid directly importing these modules in the root module.
3. Avoid Eager Imports in the Root Module
For lazy loading to work correctly, feature modules should not be eagerly imported into the AppModule or any other module. Ensure they are loaded exclusively through the routing configuration.
How do Angular modules handle preloading?
Preloading in Angular refers to the process of loading feature modules in the background after the application has initialized, without waiting for user interaction. This approach improves navigation speed while maintaining an optimized initial load time. Preloading strategies include:
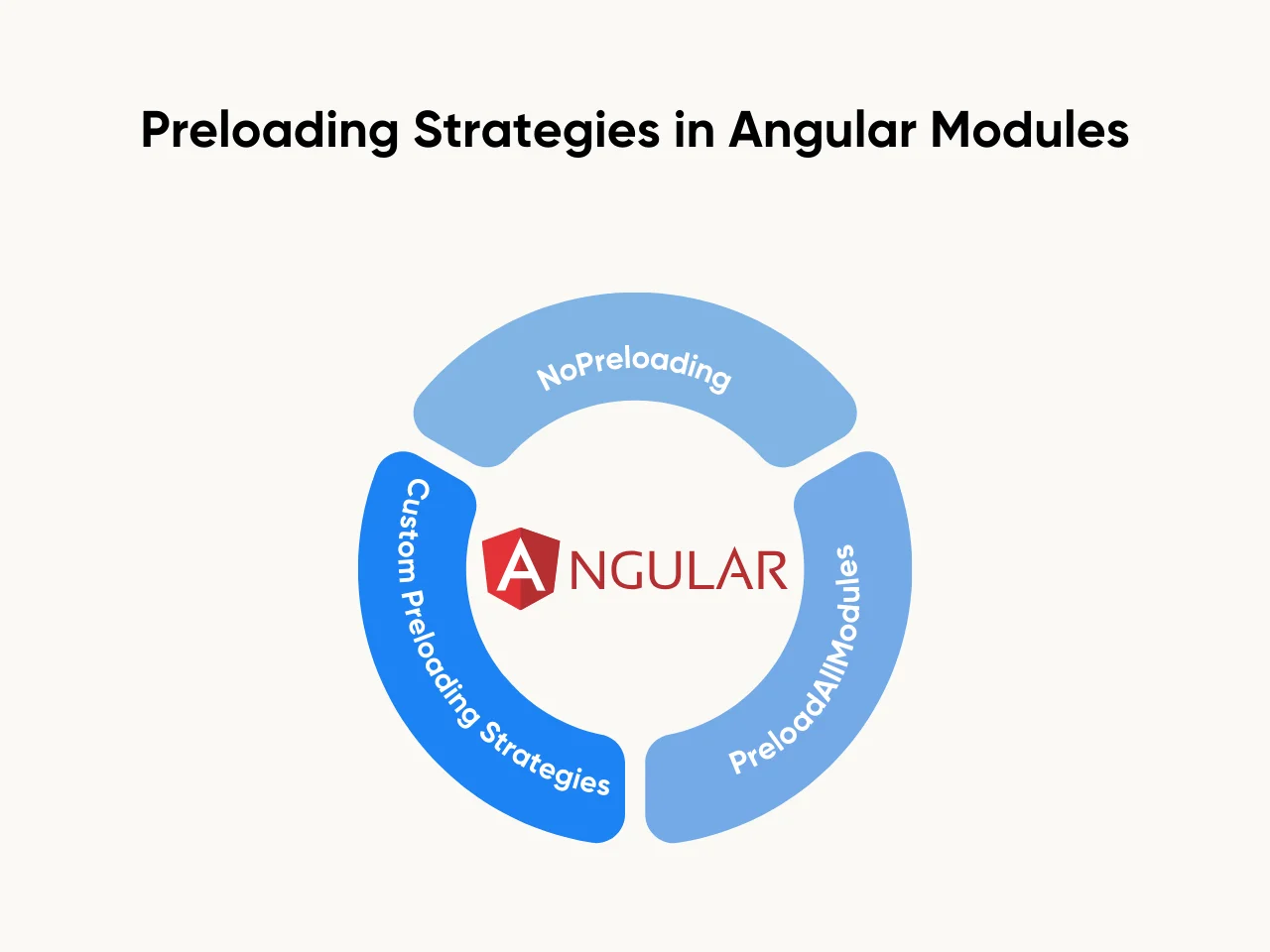
- NoPreloading: This is Angular’s default behavior, where lazy-loaded modules are not preloaded. Modules are fetched only when their routes are accessed.
- PreloadAllModules: Preloads all lazy-loaded modules in the background immediately after the application initializes, ensuring fast access to all features. This strategy is suitable for applications with ample resources and where all modules are likely to be used.
- Custom Preloading Strategies: Developers can create custom strategies by implementing the PreloadingStrategy interface. These strategies allow selective preloading of modules based on criteria like user roles or network conditions, enhancing efficiency for specific application requirements.
What is the role of the exports array in an Angular module?
In Angular, the exports array of an NgModule plays a crucial role in making components, directives, and pipes available for use in other modules. By exporting these declarations, Angular ensures that the functionality defined within a module can be shared across the application, fostering reusability and modular design.
The exports array specifies which parts of a module are accessible to other modules that import it. For example:
- A component declared in a module but not exported cannot be used in the templates of other modules.
- Only items listed in the exports array can be accessed outside the module.
For a simple example, consider a SharedModule that contains reusable UI components like buttons or headers. By adding these components to the exports array, other modules can import SharedModule and use its components without needing to redefine them:
- Shared Module: Contains components, directives, and pipes used across the application.
- Exports Array: Lists all items that should be accessible outside the module.
Angular interview questions may also include Angular Router. The Angular Router is essential for developing single page applications (SPA). It helps create a seamless user experience by loading different components based on the application's state. The router service provides navigation between views, whereas tools like the current routerState are used to determine the active route and manage the user experience effectively.
Angular’s view encapsulation and change detection mechanism are also advanced concepts that help maintain a clean separation between DOM elements and their respective styles. Angular provides AOT compiler and JIT compiler options, both of which optimize the compilation process of typescript code into efficient JavaScript code to be executed by the web browser.
Angular Universal is another advanced feature that enables server-side rendering to improve SEO and initial load performance of web pages. It can be combined with the Angular Router for faster navigation and improved user experience.
Angular provides error handling mechanisms that include error callbacks for managing errors during asynchronous operations and APIs to handle routing errors, enhancing the application's reliability.
Practical Scenarios and Problem-Solving Questions
Building scalable applications with Angular often involves navigating real-world challenges such as managing dependencies, optimizing module design, and ensuring performance. These scenarios are a key focus of Angular interview questions, testing a developer's ability to apply Angular’s core principles while maintaining a clean and modular architecture.
How do you resolve circular dependency issues between modules?
Circular dependencies in Angular occur when two or more modules depend on each other directly or indirectly, causing issues in module resolution and application stability. Resolving these dependencies is essential for maintaining clean, modular code and ensuring proper functionality.
When asked Angular interview questions, be sure to remember that the causes of circular dependencies include:
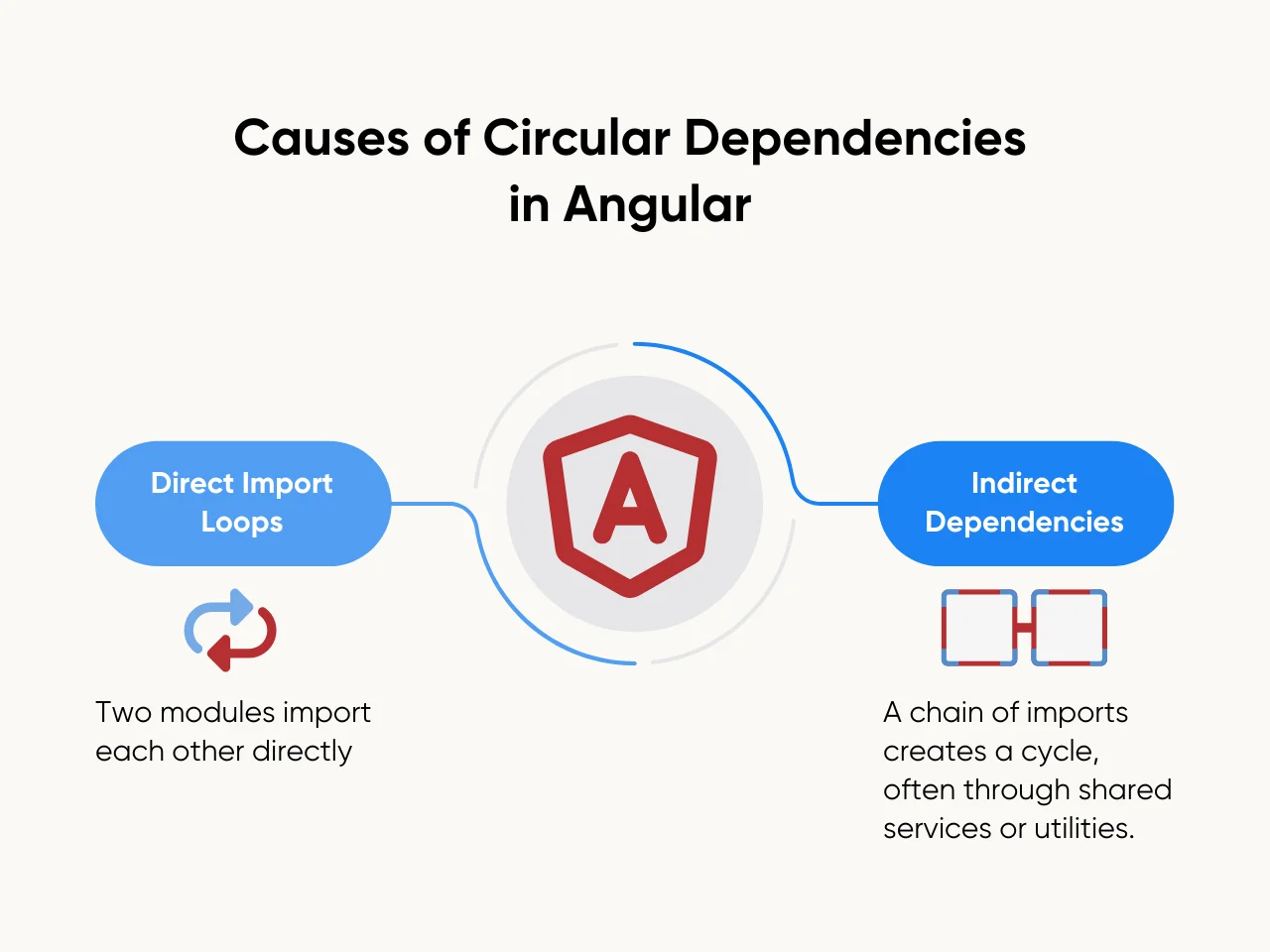
- Direct Import Loops: Two modules import each other directly.
- Indirect Dependencies: A chain of imports creates a cycle, often through shared services or utilities.
Solutions:
- Break Dependencies into a Shared Module or Service: Consolidate shared functionalities into a CoreModule or SharedModule that both modules can import, breaking the cyclic relationship and centralizing shared logic.
- Use Dependency Injection for Runtime Dependencies: Instead of static imports, use Angular’s DI system to dynamically provide services. This avoids compile-time circular references by injecting shared services where needed rather than importing their modules.
How would you optimize module imports in a large Angular application?
Adopting the following best practices ensures a cleaner architecture and reduces application load overhead:
- Keep the AppModule Lean: Limit the AppModule to core application setup, such as global services and root-level configurations. Delegate feature-specific functionalities to dedicated feature modules to avoid overloading the AppModule, simplifying debugging and improving performance.
- Leverage Lazy-Loaded Modules: Use lazy loading for feature modules to defer their loading until needed. This reduces initial load time and memory usage, ensuring resources are loaded only on demand.
- Consolidate Reusable Modules: Group commonly used components, directives, and pipes into shared or core modules. Import these modules only where necessary to avoid redundancy and maintain consistency. For instance, a SharedModule can house UI elements like buttons and headers, while a CoreModule can manage singleton services.
How do you test Angular modules?
Testing Angular modules ensures that components, directives, pipes, and services work correctly within the module’s context. A robust testing strategy isolates module dependencies, validates configurations, and verifies interactions among elements. Key strategies includ
- Use TestBed.configureTestingModule: Utilize Angular’s TestBed to configure a testing module that simulates the module’s runtime environment. This isolates dependencies and ensures all declared components, directives, and services are correctly initialized.
- Verify Module Elements Work Together: Test components to confirm they render the correct elements and handle user interactions as expected. Additionally, validate that pipes format and process data accurately within the module context. These tests ensure the module’s elements function cohesively and achieve the intended outcomes.
Behavioral and Design Questions
Behavioral and design Angular interview questions are designed to gauge your ability to apply technical knowledge to real-world scenarios. These Angular interview questions test your technical expertise and how you approach challenges, collaborate with teams, and deliver impactful solutions in practical contexts.
Describe a project where you designed a modular architecture in Angular.
When preparing for Angular interview questions, discussing your experience with modular architecture can showcase your technical expertise and problem-solving skills. A great way to structure your response is by using the STAR format: Situation, Task, Action, and Result. This method allows you to present your contributions clearly while demonstrating the impact of your work.
For example, imagine redesigning an e-commerce application using Angular. Here’s how you might structure your answer:
- Situation: The application was monolithic, with all features bundled into a single module, resulting in slow load times and poor scalability. The business required a more efficient architecture to support increasing traffic and complex features.
- Task: You were tasked with refactoring the application into a modular structure to improve performance and maintainability. Key goals included reducing load times and ensuring seamless navigation between features.
- Action: You identified core features such as product browsing, user management, and checkout, then separated these into distinct feature modules. Lazy loading was implemented for each module, ensuring they loaded only when accessed.
- Result: The changes improved page load times by 40%, enhanced scalability, and simplified future updates and feature additions.
How do you ensure maintainability and scalability in your Angular module design?
Designing Angular modules for maintainability and scalability involves adhering to best practices that simplify development, promote modularity, and prepare the application for future growth.
Strategies for effective module design include:
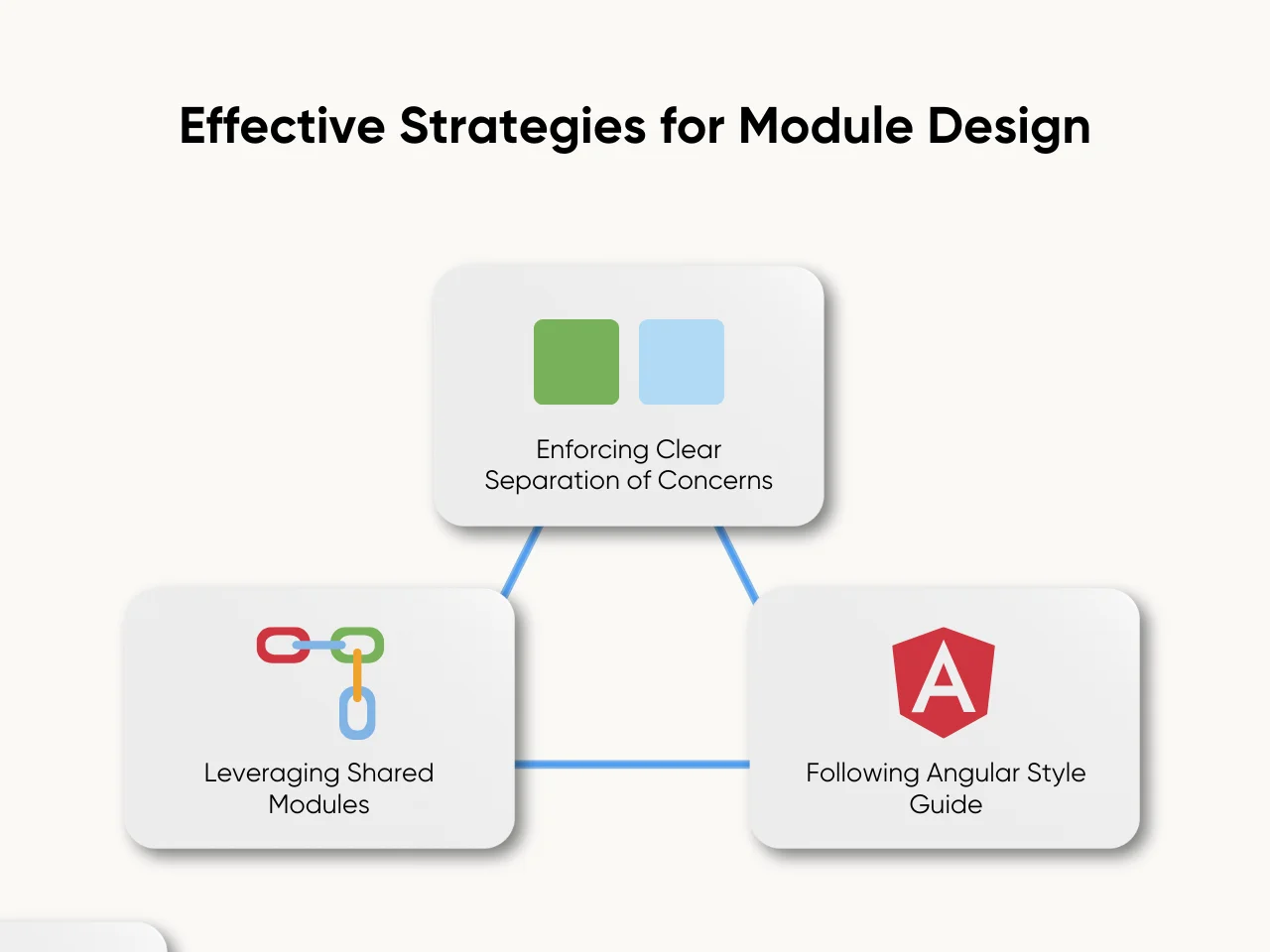
- Enforcing Clear Separation of Concerns: Organizing the application into feature-specific modules, such as UserModule for user management or ProductModule for product-related functionalities, ensures each module has a distinct purpose.
- Leveraging Shared Modules: Create shared modules for common dependencies like UI components, directives, and pipes that multiple feature modules use. This avoids duplication and promotes consistency across the application.
- Following Angular Style Guide: Adhering to Angular’s style guide provides consistent naming conventions, file data structures and coding standards. This uniformity simplifies onboarding new developers and ensures that the application scales without chaos.
Key Takeaway
Preparing for Angular interview questions should involve understanding both basic and advanced topics, from basic building blocks such as modules and lifecycle hooks to advanced concepts like Angular Universal and AOT compilation. Through mastery of these concepts, you can efficiently build and maintain web applications that are scalable, reliable, and provide a seamless user experience.
Acing Angular interview questions and answers takes more than just technical know-how; it requires a clear understanding of root modules, shared services, and scalability practices.
Whether you’re a job applicant preparing for AngularJS interview questions or a business seeking to outsource development, Aloa is here to support you. We provide resources to help developers excel in technical challenges and connect businesses with vetted teams, delivering scalable solutions through a transparent project management framework. Explore Aloa today.