Spring Boot has become a critical skill for Java application developers aiming to excel in their careers. Its ability to streamline Spring application development, especially in microservices and enterprise environments, makes it a must-have expertise. For professionals, mastering the “Spring Boot interview questions for 10 years experience” requirement is often the gateway to advancing into senior roles. Employers expect candidates to handle advanced topics like dependency injection, performance tuning, and security with confidence, making preparation essential.
At Aloa, we understand the high stakes of technical interviews. Drawing from real-world development expertise, we’ve compiled key Spring Boot interview questions for experienced developers to help you prepare effectively. Whether it’s tackling tricky architecture queries or showcasing your microservices know-how, this guide is tailored to help you stand out in competitive hiring processes.
To help you ace your interviews, we’ve prepared a comprehensive breakdown of advanced Spring Boot microservices interview questions, performance optimization techniques, and solutions for dependency injection challenges. Packed with actionable examples and expert advice, this resource will equip you with the knowledge and confidence to secure your next role.
Let’s get started!
Core Spring Boot Architecture and Design Questions
Spring Boot streamlines application development by introducing powerful features like auto-configuration, embedded servers, and opinionated defaults. These features are essential knowledge points in Spring Boot interview questions, as they eliminate the need for extensive manual configurations, making building web applications quickly easier for developers.
How does Spring Boot simplify application development compared to traditional Spring?

Spring Boot is a Java-based framework for Rapid Application Development and building stand-alone microservices. It streamlines development with auto-configuration, embedded servers like Tomcat and Jetty, and opinionated defaults. This allows developers to focus on business features instead of boilerplate code while creating production-ready applications efficiently.
- Auto-Configuration: Spring Boot intelligently configures the application based on its dependencies. For example, if the classpath contains Spring Boot starter web or spring-boot-starter-web, the Spring framework automatically sets up a web server, a Spring MVC architecture, and key components, eliminating the need for XML or Java-based configuration.
- Embedded Servers: With built-in support for servers like an embedded Tomcat server or Jetty, Spring Boot allows applications to run as standalone JAR files. This removes the dependency on external server installations and simplifies deployment and testing processes.
- Opinionated Defaults: The framework provides pre-configured defaults for logging, database connections, and more. Developers can override these defaults as needed, but they save significant development time by providing a sensible starting point for most use cases.
Additionally, Spring Boot provides tools like the Spring Boot Starter POM and Spring Boot CLI to further streamline the development process:
- Spring Boot Starter POM: These Starter POMs are pre-configured dependencies for functions like database, security, Maven configuration, etc. They simplify dependency management and ensure compatibility among libraries.
- Spring Boot CLI (Command Line Interface): This command-line tool is designed for managing dependencies, creating projects, and running applications efficiently, especially for rapid prototyping.
The entry point of a Spring Boot application is the class annotated with @SpringBootApplication, which contains the main method. This annotation simplifies setup by combining configurations like component scanning and auto-configuration, drastically reducing complexity. It enables quick setup for applications like web apps, allowing them to run independently with minimal effort.
What is Spring Boot’s auto-configuration mechanism, and how does it work?
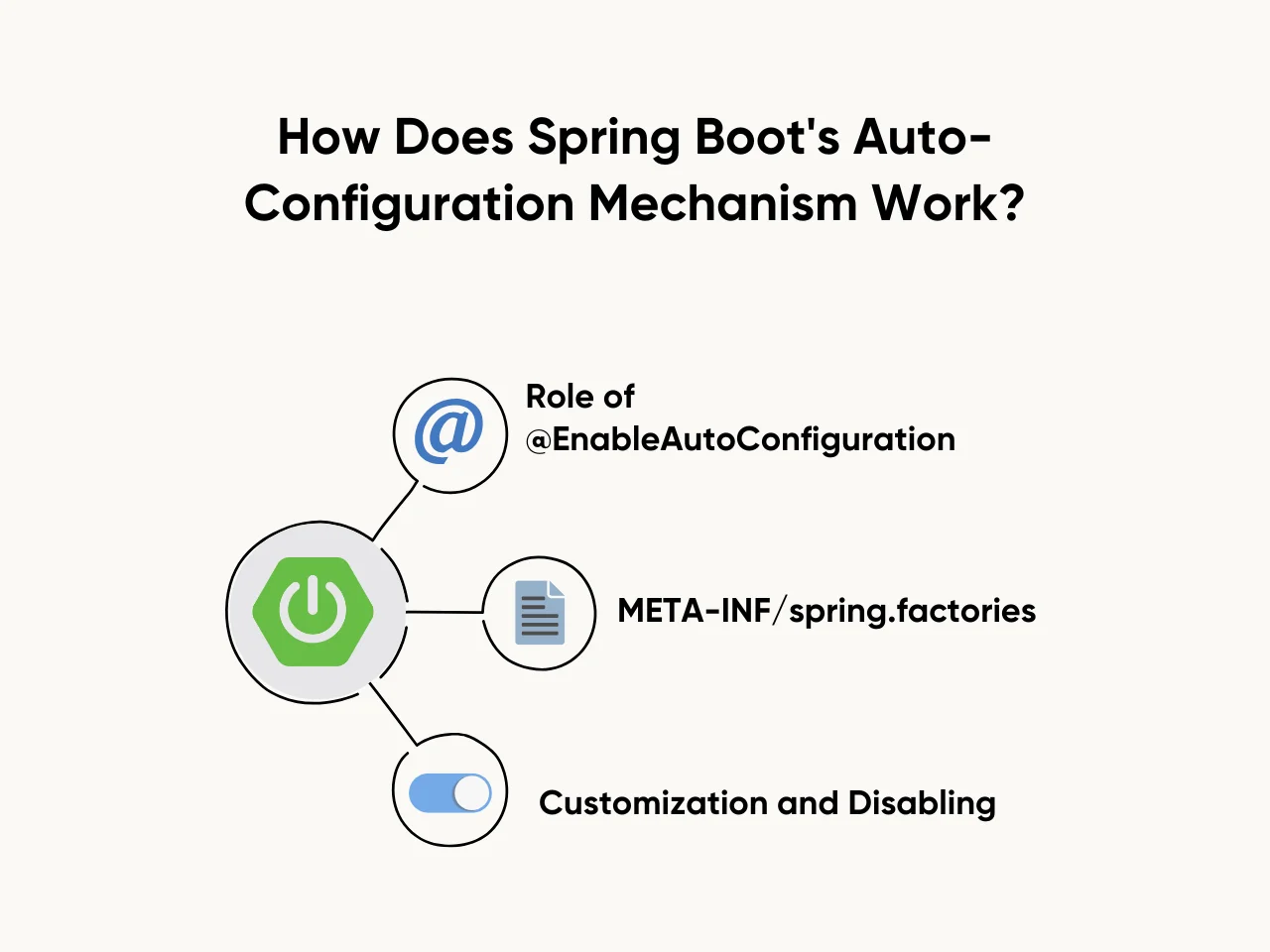
Spring Boot’s auto-configuration mechanism simplifies application setup by automatically configuring beans and application settings based on the dependencies in the classpath. Such features of Spring Boot eliminate the need for extensive manual configuration and are a frequent topic in Spring Boot interview questions.
- Role of @EnableAutoConfiguration: The @EnableAutoConfiguration annotation directs Spring Boot to configure beans automatically. It uses the classpath, existing configurations, and the META-INF/spring.factories file to determine which configurations to apply.
- META-INF/spring.factories: This file lists the auto-configuration classes that Spring Boot can use. It ensures that the application starts with the necessary configurations without requiring developer input.
- Customization and Disabling: Developers can customize auto-configuration by excluding specific classes using the exclude attribute in @EnableAutoConfiguration or @SpringBootApplication.
How do you design a modular application in Spring Boot?
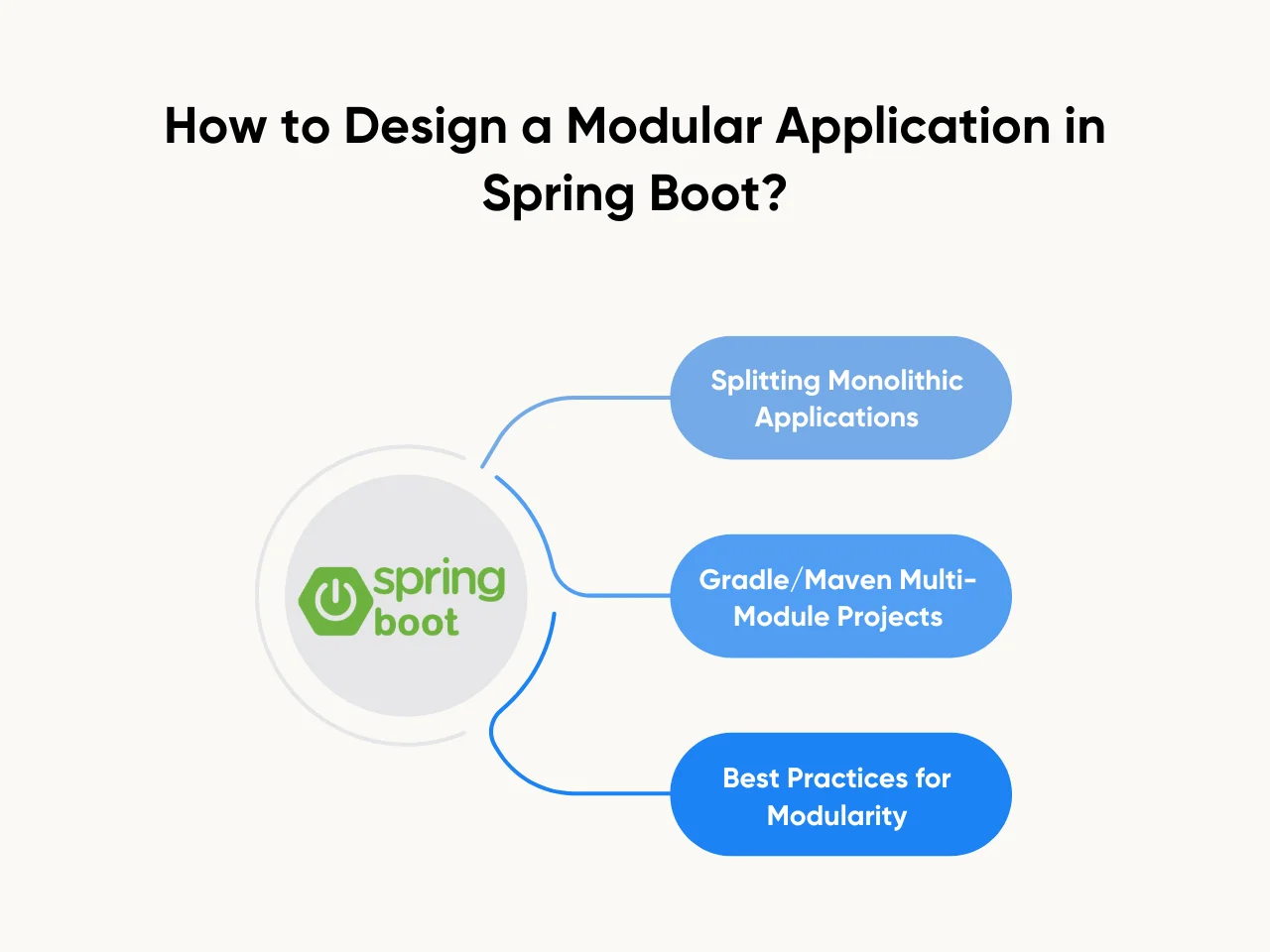
Designing modular applications in Spring Boot ensures scalability and maintainability, especially for enterprise-level systems. This is a common area of inquiry in Springboot interview questions.
- Splitting Monolithic Applications: Monolithic architectures can be modularized by creating distinct modules for each feature or domain. These modules use multiple @Configuration classes to manage their dependencies and logic independently.
- Gradle/Maven Multi-Module Projects: Gradle and Maven support modular applications by organizing modules under a parent project. Gradle enables separate builds while sharing resources, and Maven centralizes dependencies using a parent POM for easier management.
- Best Practices for Modularity: Create feature-specific modules for distinct functionalities (e.g., user management, payments), use shared modules for reusable components, and apply lazy loading to optimize performance in distributed systems with Spring Cloud.
Advanced Dependency Injection and Bean Management
Dependency injection (DI) is a cornerstone of Spring Boot, enabling developers to write modular, testable, and maintainable applications. Advanced topics like stereotypes and handling circular dependencies frequently appear in Spring Boot interview questions for experienced developers.
What is the difference between @Component, @Service, @Repository, and @Controller annotations?
Spring Boot leverages stereotype annotations to streamline dependency injection and component management. These annotations, each serving specific roles, are crucial for building layered architectures:
- @Component: A generic stereotype used for any Spring bean component. It is the foundation for more specific Spring Boot annotations.
- @Service: Marks a class in the service layer where business logic is performed. For example, a UserService handles user-related operations using data mapped to JPA entities. The repository layer manages CRUD operations for Spring Data REST APIs, ensuring smooth database interaction.
- @Repository: Specializes in data access logic, such as interacting with databases. It also provides exception translation for persistence-related errors.
- @Controller: Used in the web layer to handle HTTP requests and direct responses, typically in an MVC context.
These annotations ensure clean separation of concerns, promoting scalability and maintainability in Spring Boot applications with their Spring ecosystem.
How do you handle circular dependencies in Spring Boot?
Circular dependencies occur when two or more beans depend on each other directly or indirectly, leading to an infinite loop during initialization. This is a critical topic in advanced Spring Boot interview questions, as it tests a developer's ability to resolve complex DI challenges.
Circular dependencies typically arise from tight coupling between components. For example, a ServiceA might depend on ServiceB, while ServiceB references ServiceA.
To address this, developers can adopt several solutions:
- Refactor to Break Dependency Chains: Redesign the architecture to decouple tightly coupled components. Introducing an intermediary layer or service often resolves the issue.
- @Lazy Annotation: Apply @Lazy to delay bean initialization until it is required, breaking the initialization loop.
- ObjectFactory: Use ObjectFactory to dynamically resolve dependencies at runtime, ensuring deferred initialization.
How do you manage bean scopes in Spring Boot?
Spring Boot supports multiple bean scopes to control the lifecycle and visibility of beans in the application context. Choosing the appropriate scope ensures efficient resource utilization and tailored functionality.
In answering Spring Boot interview questions, remember these common bean scopes:
- Singleton: The default scope; a single bean instance is created and shared throughout the application context.
- Prototype: Creates a new bean instance each time it is requested.
- Request: A new bean instance is created for each HTTP request (applicable in web applications).
- Session: Specific to different environments, a new bean instance is created for each user session (also specific to web applications).
For example, to define a bean's scope explicitly, use the @Scope annotation. For instance, setting a bean as prototype-scoped ensures that every request for the bean creates a new instance, which is useful for transient data handling.
Mastering bean scopes allows developers to optimize memory usage and application performance.
Microservices and Distributed System Design
Spring Boot simplifies the development and deployment of microservices through a variety of integrated tools and components. Its flexibility makes it an ideal framework for building distributed systems, a topic frequently explored in Spring Boot interview questions.
How does Spring Boot facilitate microservices architecture?
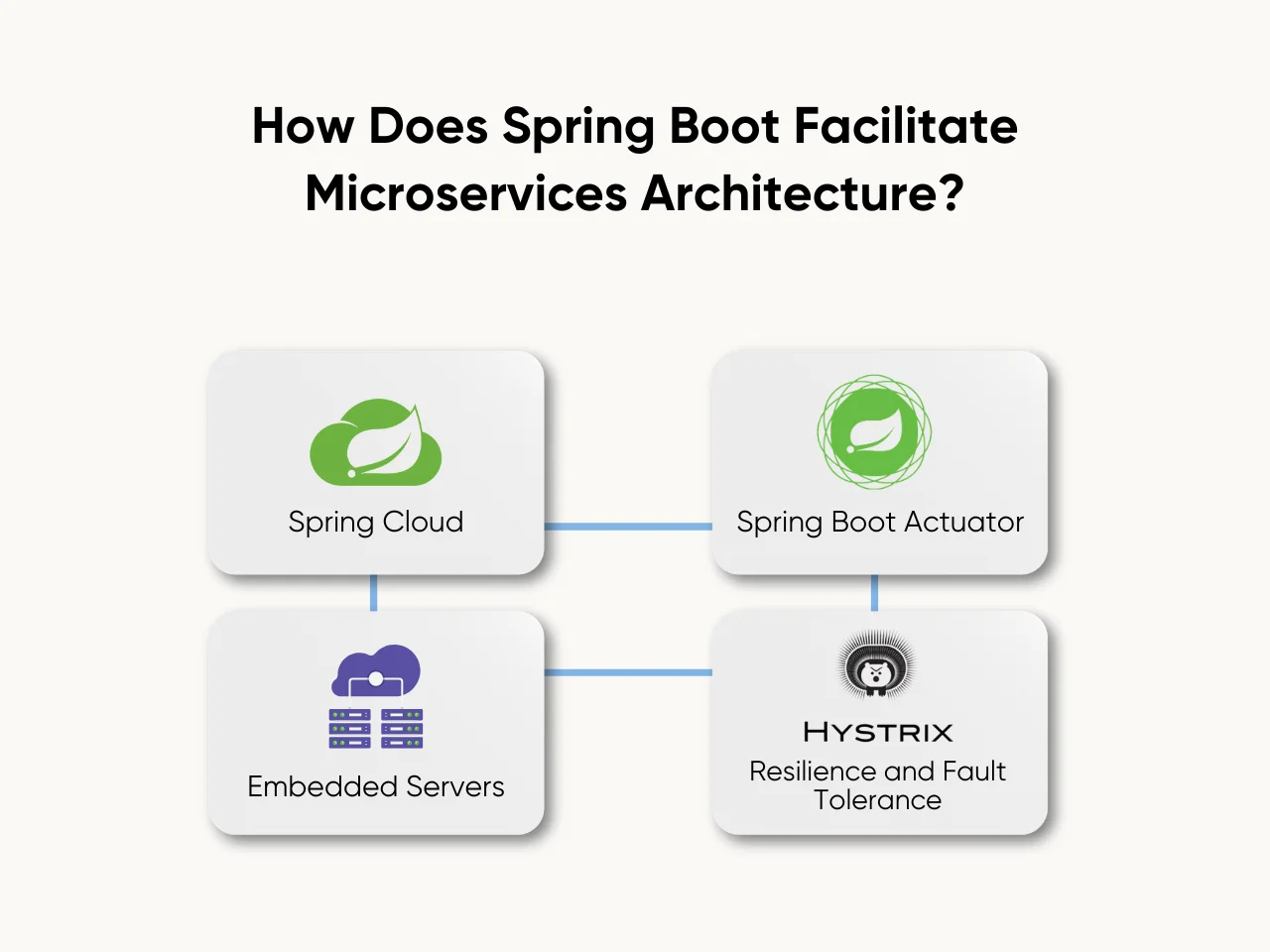
Spring Boot offers comprehensive tools and libraries for designing microservices, addressing common challenges in distributed system design:
- Spring Cloud: Provides tools like Eureka for service discovery, Ribbon for client-side load balancing, and Zuul or Spring Cloud Gateway for API management. These components simplify inter-service communication and routing.
- Spring Boot Actuator: Enables monitoring and health checks of microservices. Actuator endpoints provide metrics, health statuses, and system information, helping teams maintain system reliability.
- Embedded Servers: Spring Boot applications come with embedded servers like Tomcat or Jetty, enabling quick deployments without external configurations.
- Resilience and Fault Tolerance: Tools like Hystrix implement circuit breaker patterns to handle service failures gracefully.
These features allow developers to design scalable, easily deployable microservices that integrate seamlessly with modern containerization platforms like Docker and Kubernetes.
What is Spring Boot’s support for distributed tracing?
Distributed tracing enables monitoring and troubleshooting requests as they flow through multiple microservices. Spring Boot integrates tools like Spring Cloud Sleuth and Zipkin to provide seamless distributed tracing capabilities:
- Spring Cloud Sleuth: Sleuth adds unique trace and span IDs to requests and logs, ensuring traceability across microservices. These IDs propagate with requests, helping identify bottlenecks and failures.
- Zipkin for Visualizing Traces: Zipkin collects trace data from Sleuth-enabled applications and provides a user-friendly dashboard for analyzing request flows and identifying latency issues.
For example, implementing distributed tracing in Spring Boot involves the following steps:
- Add the Sleuth and Zipkin dependencies to your Spring Boot project.
- Sleuth automatically generates trace and span IDs without requiring additional code.
- To create custom spans, use Sleuth’s APIs to annotate key events or process steps.
Distributed tracing enhances observability and ensures efficient microservices operations.
How do you handle configuration management in Spring Boot microservices?

Managing configurations across multiple microservices can be challenging. Spring Boot addresses this with the Spring Cloud Config Server, providing a centralized solution for configuration management. Additionally, its externalized configuration feature enables a single application codebase to run across different environment variables by storing configurations separately, ensuring flexibility and ease of maintenance.
- Centralized Configurations: Store configuration files in a version-controlled repository (e.g., Git) and access them through the Config Server. This ensures consistent configurations across environments.
- Externalized Configurations: Use the application.yml or application.properties property files for individual microservices. Configurations can include environment-specific properties such as database URLs, API keys, or feature toggles.
For example, you can set up configuration management with the following steps:
- Create a Config Server: Add the necessary dependencies for Spring Cloud Config to your project.
- Connect to a Git Repository: Point the server to a Git repository that stores your configuration files.
- Client Microservices Integration: Configure client microservices to connect to the Config Server and retrieve their configurations dynamically at runtime.
This centralized approach simplifies configuration management, enhances scalability, and reduces the likelihood of errors during deployments.
Performance Optimization and Scalability Questions
Spring Boot provides several built-in features and tools to enhance performance and scalability. These optimizations help applications handle high-concurrency environments, reduce latency, and efficiently utilize resources.
How do you tune Spring Boot applications for high performance?
Optimizing a Spring Boot application involves fine-tuning processes and implementing strategies to ensure faster response times and better resource management. Here are some key techniques:
- Enable Asynchronous Processing: Use the @Async annotation to execute methods asynchronously, freeing up the main thread for other tasks. This is particularly useful for long-running or non-blocking operations.
- Optimize Database Interactions: Improve database performance by leveraging Spring Data repositories or Spring Data JPA for efficient ORM (Object Relational Mapping). Fine-tune queries using custom JPQL or native SQL and enable connection pooling to handle concurrent requests effectively.
- Simplify ORM with Hibernate: Utilize Hibernate, a JPA implementation, to efficiently map, store, and retrieve data between Java objects and relational databases, ensuring seamless application data management.
- Utilize Caching: Implement caching using Spring Cache abstraction with providers like Redis, Ehcache, or Caffeine. Annotations such as @Cacheable and @CacheEvict store frequently accessed data, significantly reducing response times.
Spring Boot applications can handle higher loads, ensure faster response times, and maintain stability under stress by implementing these strategies.
How do you handle large-scale data processing in Spring Boot?
Spring Boot offers Spring Batch, a robust framework for batch processing of large datasets. It enables the processing of millions of records efficiently through features like job scheduling and chunk-based processing:
- Spring Batch for ETL Pipelines: Use Spring Batch to build ETL (Extract, Transform, Load) pipelines. It processes data in chunks, ensuring efficient memory usage and performance.
- Job Scheduling: Leverage Spring Batch’s scheduling mechanisms to manage complex workflows, like processing data at scheduled intervals or handling data migrations.
For example, you can design a pipeline that reads data from a CSV file, applies transformation logic, and writes the processed data to a database. Using chunk processing, the application processes records in smaller batches, reducing memory usage and improving performance.
How do you optimize Spring Boot for high-concurrency environments?
To support high-concurrency environments, Spring Boot provides several strategies for optimizing resource utilization and managing heavy workloads.
- Connection Pooling with HikariCP: Spring Boot integrates HikariCP as the default connection pool, known for its speed and low latency. Properly configure the pool size and timeout settings to handle simultaneous database connections effectively.
- Thread Pools for @Async Methods: Use the @Async annotation with custom thread pools to offload tasks to background threads. Configure the pool size and task queue capacity in the TaskExecutor bean to manage concurrent requests seamlessly.
- Optimize Session Management: Implement distributed session management with tools like Spring Session and storage solutions like Redis. This approach ensures consistent session handling across multiple instances in a clustered environment.
Spring Boot applications can achieve high scalability, reduced latency, and improved user experience during peak loads by combining these strategies.
Security and Testing in Spring Boot
Spring Boot provides a robust foundation for developing secure and well-tested applications. By leveraging tools like Spring Security and effective exception handling mechanisms, developers can build reliable and secure APIs.
How do you implement authentication and authorization in Spring Boot?
Spring Boot simplifies authentication and authorization through integration with Spring Security, offering flexibility with methods like OAuth2 and JWT for token-based authentication.
Spring Security supports secure API development through token-based authentication methods like OAuth2 and JWT (JSON Web Tokens). OAuth2 is widely used for securing APIs by providing robust mechanisms for token issuance and validation. Similarly, JWT offers a lightweight and secure approach for transmitting information between parties, encapsulated in a digitally signed token that ensures data integrity and authenticity.
For example, role-based access control can be implemented using the @PreAuthorize annotation. By annotating methods with access restrictions based on roles or conditions, you can ensure secure API endpoints. For instance, a method annotated with @PreAuthorize("hasRole('ADMIN')") restricts access to users with the admin role.
What is the role of @RestControllerAdvice in error handling?
@RestControllerAdvice is a specialized annotation in Spring Boot for centralizing exception handling in RESTful web services applications. It combines @ControllerAdvice and @ResponseBody, making it ideal for global error management in APIs.
Centralized exception handling in Spring Boot can be achieved by defining methods annotated with @ExceptionHandler. These methods are designed to handle specific exceptions across multiple controllers, streamlining error management throughout the application. This approach reduces code duplication by consolidating exception handling in a single location and ensures consistent and standardized error responses, enhancing the overall reliability and maintainability of the API.
For example, you can create a method in a class annotated with @RestControllerAdvice to customize error responses for validation errors. When a MethodArgumentNotValidException is thrown, the method formats the error details into a JSON response, improving API usability and client debugging.
How do you test a Spring Boot application?
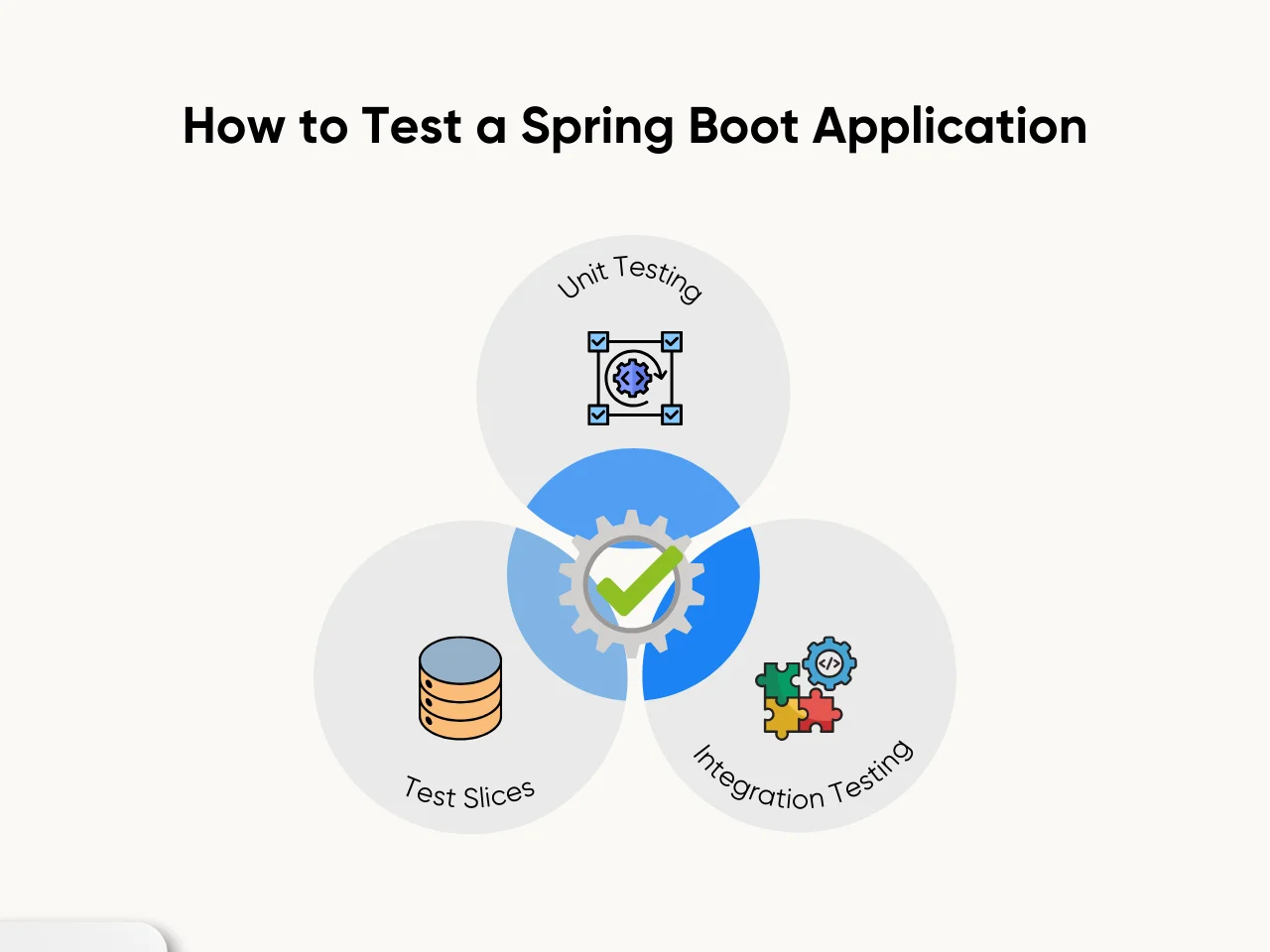
Spring Boot provides tools for unit and integration testing, as well as targeted test slices for specific layers of the application. These testing strategies are often discussed in Spring Boot interview questions, highlighting their importance in building reliable applications:
- Unit Testing with JUnit and Mockito: Use JUnit to structure test cases and Mockito to mock dependencies for isolated service-level testing.
- Integration Testing with TestRestTemplate and MockMvc: TestRestTemplate is ideal for testing REST APIs, while MockMvc simulates HTTP requests to controllers without starting the server.
- Test Slices for Focused Testing: Annotations like @WebMvcTest focus on testing the web layer, while @DataJpaTest configures an in-memory database for validating JPA repository operations.
For example, you can use MockMvc to simulate an HTTP request to a controller endpoint and validate the response. With @DataJpaTest, you can test repository methods against an in-memory database to ensure query correctness.
These techniques ensure comprehensive testing of Spring Boot applications with minimal overhead, making them a valuable topic to explore when preparing for Spring interview questions.
Key Takeaway
Spring Boot interview questions can feel like navigating a maze of architecture, dependency management, and performance optimization. Succeeding in these interviews means not just knowing the theory but proving your expertise through real-world examples.
Are you ready to tackle circular dependencies, high-concurrency optimization, or distributed tracing? Mastering these advanced Spring Boot topics requires deep knowledge and hands-on experience. How confident are you in showcasing your expertise and leaving a lasting impression?
Boost your skills and ace tough Spring Boot interview questions with Aloa’s curated guide. Packed with actionable insights and real-world examples, it’s your path to standing out as a top candidate. Visit Aloa Blog to learn more on how to elevate your career.